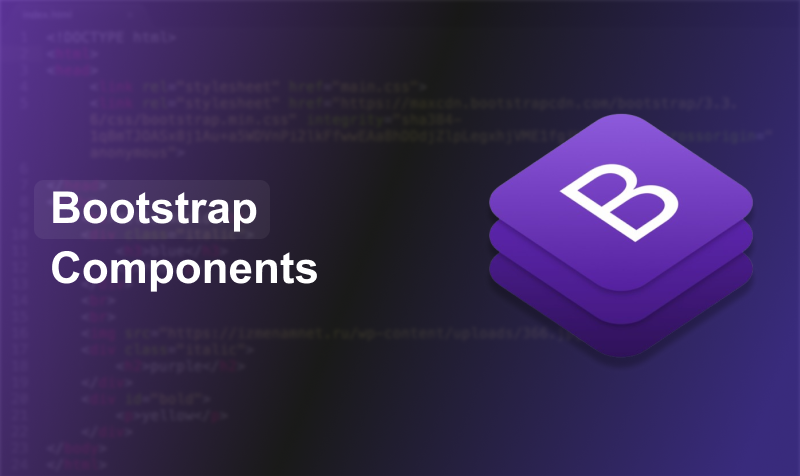
Button
Bootstrap button components provide versatile styles and sizes for easy customization and consistent design in web projects.
<button type="button" class="btn btn-primary">Primary Button</button>
Button group
Button group components allow you to group a series of buttons together on a single line. It is used to create toolbars, segmented controls, or combined actions.
<div class="btn-group" role="group" aria-label="Basic example">
<button type="button" class="btn btn-secondary">Download</button>
<button type="button" class="btn btn-secondary">Buy Now</button>
<button type="button" class="btn btn-secondary">Click Here</button>
</div>
Close Button
Bootstrap close button components provide a simple and consistent way to add dismiss buttons to alerts, modals, and other elements.
<button type="button" class="btn-close" aria-label="Close"></button>
Avatar
Avatar components offer a simple way to display user profile images with consistent styling and optional customization.
<img src="avatar.png" alt="User Avatar" class="rounded-circle" width="50" height="50">
Avatar Group
Avatar group components allow you to display multiple avatars together in a compact and organized manner. It can be customized with various sizes and shapes for a consistent look.
<div class="avatar-group">
<img src="avatar1.png" alt="User 1" class="rounded-circle" width="40" height="40">
<img src="avatar2.png" alt="User 2" class="rounded-circle" width="40" height="40">
<img src="avatar3.png" alt="User 3" class="rounded-circle" width="40" height="40">
</div>
Badge
Badge components are versatile, small count or labeling elements used to highlight new or unread items, statuses, or notifications.
<button type="button" class="btn btn-primary">
New <span class="badge bg-secondary">4</span>
</button>
Table
Table components provide a flexible and responsive way to display data in a tabular format with built-in styles for enhanced readability.
<table class="table table-striped">
<thead>
<tr>
<th scope="col">Sr.No</th>
<th scope="col">Name</th>
<th scope="col">Age</th>
<th scope="col">City</th>
</tr>
</thead>
<tbody>
<tr>
<th scope="row">1</th>
<td>Emma</td>
<td>28</td>
<td>New York</td>
</tr>
<tr>
<th scope="row">2</th>
<td>Joy</td>
<td>30</td>
<td>Los Angeles</td>
</tr>
</tbody>
</table>
Alerts
Alerts are used to convey important messages or feedback to users.
<div class="alert alert-success" role="alert">
Your work have been saved successfully!
</div>
Modals
Modals are interactive components for displaying content in a pop-up dialog box, which can be triggered by various actions.
<!-- Button trigger modal -->
<button type="button" class="btn btn-primary" data-bs-toggle="modal" data-bs-target="#exampleModal">
Demo modal
</button>
<!-- Modal -->
<div class="modal fade" id="exampleModal" tabindex="-1" aria-labelledby="exampleModalLabel" aria-hidden="true">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title" id="exampleModalLabel">Modal title</h5>
<button type="button" class="btn-close" data-bs-dismiss="modal" aria-label="Close"></button>
</div>
<div class="modal-body">
Content for the modal goes here.
</div>
<div class="modal-footer">
<button type="button" class="btn btn-secondary" data-bs-dismiss="modal">Close</button>
<button type="button" class="btn btn-primary">Save changes</button>
</div>
</div>
</div>
</div>
Placeholder
Placeholders are used to create loading states for components, giving a visual cue while content is being loaded.
<div class="placeholder-glow"><span class="placeholder col-12"></span></div>
Popover
Popovers are similar to tooltips but can contain more complex content like headings, paragraphs, and images. It overlay elements used to provide additional content or information when a user hovers over or clicks on a target element.
<button type="button" class="btn btn-lg btn-danger" data-bs-toggle="popover" title="Popover title" data-bs-content="And here's some new content. It's very engaging. Right?">
Click to toggle popover
</button>
<script>
var popoverTriggerList = [].slice.call(document.querySelectorAll('[data-bs-toggle="popover"]'))
var popoverList = popoverTriggerList.map(function (popoverTriggerEl) {
return new bootstrap.Popover(popoverTriggerEl)
})
</script>
Progress bar
Progress bars are used to visualize the progression of a task, displaying a horizontal bar that fills up as the task advances.
<div class="progress">
<div class="progress-bar" role="progressbar" style="width: 70%;" aria-valuenow="70" aria-valuemin="0" aria-valuemax="100">70%</div>
</div>
Spinner
Spinners show that something is loading, giving a visual clue while waiting for content or a task to finish. They come in different styles and can be customized easily.
<div class="spinner-border" role="status">
<span class="visually-hidden">Loading...</span>
</div>
Tooltip
Tooltip are small pop-up messages that appear when a user hovers over or focuses on an element.
<button type="button" class="btn btn-secondary" data-bs-toggle="tooltip" data-bs-placement="top" title="Tooltip on top">
Hover over me
</button>
<script>
var tooltipTriggerList = [].slice.call(document.querySelectorAll('[data-bs-toggle="tooltip"]'))
var tooltipList = tooltipTriggerList.map(function (tooltipTriggerEl) {
return new bootstrap.Tooltip(tooltipTriggerEl)
})
</script>
Checkbox
Checkbox are used to create styled, customizable checkboxes for forms, allowing users to select one or multiple options.
<div class="form-check">
<input class="form-check-input" type="checkbox" value="" id="flexCheckDefault">
<label class="form-check-label" for="flexCheckDefault">
Default checkbox
</label>
</div>
Dropdown
Dropdowns are used to create toggleable menus that display a list of links or actions when clicked.
<div class="dropdown">
<button class="btn btn-secondary dropdown-toggle" type="button" id="dropdownMenuButton" data-bs-toggle="dropdown" aria-expanded="false">
Menu Name
</button>
<ul class="dropdown-menu" aria-labelledby="dropdownMenuButton">
<li><a class="dropdown-item" href="#">Item 1</a></li>
<li><a class="dropdown-item" href="#"> Item 2</a></li>
<li><a class="dropdown-item" href="#"> Item 3</a></li>
</ul>
</div>
Form
Form components are used to create well-structured and styled forms with various input fields, labels, and controls.
<form>
<div class="mb-3">
<label for="name" class="form-label">Name</label>
<input type="text" class="form-control" id="name">
</div>
<div class="mb-3">
<label for="email" class="form-label">Email</label>
<input type="email" class="form-control" id="email">
</div>
<button type="submit" class="btn btn-primary">Submit</button>
</form>
Floating Label
Floating labels are a design feature where the label of an input field moves above the field when it is focused or contains a value. It provide a clean and space-efficient way to display input labels.
<div class="form-floating">
<input type="text" class="form-control" id="floatingInput" placeholder="Your name">
<label for="floatingInput">Name</label>
</div>
Check Out – Bootstrap Alternatives
Range Sliders
It used to create interactive sliders that allow users to select a value or range by dragging a handle along a track.
<label for="customRange1" class="form-label">Example range</label>
<input type="range" class="form-range" id="customRange1">
Switch
Switch are toggle controls that let users switch between two states, such as “on” and “off,” in a visually distinct manner.
<div class="form-check form-switch">
<input class="form-check-input" type="checkbox" id="flexSwitchCheckDefault">
<label class="form-check-label" for="flexSwitchCheckDefault">Default switch checkbox input</label>
</div>
Breadcrumb
Breadcrumb are used to display a navigation path, helping users understand their current location within a website’s hierarchy.
<nav aria-label="breadcrumb">
<ol class="breadcrumb">
<li class="breadcrumb-item"><a href="#">Product</a></li>
<li class="breadcrumb-item"><a href="#">Blog</a></li>
<li class="breadcrumb-item active" aria-current="page">Guide</li>
</ol>
</nav>
Navbar
Navbar are flexible navigation components used to create responsive and customizable navigation bars for websites.
<nav class="navbar navbar-expand-lg navbar-light bg-light">
<a class="navbar-brand" href="#">Brand</a>
<button class="navbar-toggler" type="button" data-bs-toggle="collapse" data-bs-target="#navbarNav" aria-controls="navbarNav" aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse" id="navbarNav">
<ul class="navbar-nav">
<li class="nav-item">
<a class="nav-link active" aria-current="page" href="#">Home</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">Features</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">Pricing</a>
</li>
</ul>
</div>
</nav>
Pagination
Pagination components provide a way to navigate through a series of content, such as pages in a multi-page document or a list of items.
<nav aria-label="Page navigation example">
<ul class="pagination">
<li class="page-item"><a class="page-link" href="#">Previous</a></li>
<li class="page-item"><a class="page-link" href="#">1</a></li>
<li class="page-item"><a class="page-link" href="#">2</a></li>
<li class="page-item"><a class="page-link" href="#">3</a></li>
<li class="page-item"><a class="page-link" href="#">Next</a></li>
</ul>
</nav>
Scrollspy
Scrollspy automatically updates navigation links based on the scroll position, indicating which section of a page is currently visible.
<body data-bs-spy="scroll" data-bs-target="#navbar-example" data-bs-offset="0" tabindex="0">
<nav id="navbar-example" class="navbar navbar-light bg-light">
<ul class="nav nav-pills">
<li class="nav-item">
<a class="nav-link" href="#section1">Section 1</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#section2">Section 2</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#section3">Section 3</a>
</li>
</ul>
</nav>
<div style="height: 200px; overflow: auto;">
<h4 id="section1">Section 1</h4>
<p>Section 1 Content...</p>
<h4 id="section2">Section 2</h4>
<p> Section 2 Content...</p>
<h4 id="section3">Section 3</h4>
<p> Section 3 Content...</p>
</div>
</body>
Accordions
Accordions are collapsible content containers that allow users to toggle between hiding and showing sections of content. Accordions makes easier to manage large amounts of information.
<div class="accordion" id="accordionExample">
<div class="accordion-item">
<h2 class="accordion-header" id="headingOne">
<button class="accordion-button" type="button" data-bs-toggle="collapse" data-bs-target="#collapseOne" aria-expanded="true" aria-controls="collapseOne">
Accordion Item #1
</button>
</h2>
<div id="collapseOne" class="accordion-collapse collapse show" aria-labelledby="headingOne" data-bs-parent="#accordionExample">
<div class="accordion-body">
This is the content for the first accordion item.
</div>
</div>
</div>
</div>
Carousels
Carousels are dynamic, responsive components that cycle through a series of images or content. It offers a visually engaging way to display multiple items in a compact space.
<div id="carouselExample" class="carousel slide" data-bs-ride="carousel">
<div class="carousel-inner">
<div class="carousel-item active">
<img src="https://via.placeholder.com/800x400" class="d-block w-100" alt="Slide 1">
</div>
<div class="carousel-item">
<img src="https://via.placeholder.com/800x400" class="d-block w-100" alt="Slide 2">
</div>
<div class="carousel-item">
<img src="https://via.placeholder.com/800x400" class="d-block w-100" alt="Slide 3">
</div>
</div>
<button class="carousel-control-prev" type="button" data-bs-target="#carouselExample" data-bs-slide="prev">
<span class="carousel-control-prev-icon" aria-hidden="true"></span>
<span class="visually-hidden">Previous</span>
</button>
<button class="carousel-control-next" type="button" data-bs-target="#carouselExample" data-bs-slide="next">
<span class="carousel-control-next-icon" aria-hidden="true"></span>
<span class="visually-hidden">Next</span>
</button>
</div>
Empty State
Empty state components are used to display a placeholder message or image when there’s no data to show. It guides users on what actions to take next.
<div class="text-center">
<img src="https://via.placeholder.com/150" class="mb-4" alt="No data">
<p>No data available. Please add new items.</p>
<button class="btn btn-primary">Add Item</button>
</div>
Image
Image components provide responsive and customizable image styling options, making it easy to handle images of various sizes and shapes within a layout.
<img src="https://via.placeholder.com/150" class="img-fluid rounded" alt="Responsive image">
Layout
Layout components provide a flexible and responsive grid system, allowing you to create complex layouts with ease.
<div class="container">
<div class="row">
<div class="col-sm">
Column 1
</div>
<div class="col-sm">
Column 2
</div>
<div class="col-sm">
Column 3
</div>
</div>
</div>
Link
Link components provide styles and classes to enhance the appearance and behavior of hyperlinks. It also ensuring they integrate seamlessly with the rest of your design.
<a href="#" class="link-primary">Primary link</a>
Leave a Reply